1.1. The simplest example¶
Ingenico terminal troubleshooting Terminal playing up? Some of the most common issues and how to sort them are listed below. Terminal not turning on. Download input device drivers or install DriverPack Solution software for driver scan and update. To change the date: input 6 digits for the two-digit day, two-digit month, and two-digit year, then press Enter. Be careful when entering the date and time, as an invalid year may render the terminal inoperable. To change the time: Use a military time format to input 4 total digits, 2 for the hour and 2 for the minute, then press Enter. Card reader in Device Manager. Open the Device Manager in Windows 10 and look for a device called Memory technology devices. If this device is absent, your PC or laptop doesn’t have a card reader. If the device is present, expand it to view the name of your card reader. The Ingenico iSMP4 is a lightweight, Wi-Fi connected device that makes it easy to accept payments on the go, including MSR (magnetic stripe), EMV (chip), and NFC (contactless) payments. To ensure that your terminal is successfully connected to your network, you need to power on your terminal using the on/off and wake/sleep button on the right.
Here comes a very simple example of an input device driver. The device hasjust one button and the button is accessible at i/o port BUTTON_PORT. Whenpressed or released a BUTTON_IRQ happens. The driver could look like:
1.2. What the example does¶
First it has to include the <linux/input.h> file, which interfaces to theinput subsystem. This provides all the definitions needed.
In the _init function, which is called either upon module load or whenbooting the kernel, it grabs the required resources (it should also checkfor the presence of the device).
Then it allocates a new input device structure with input_allocate_device()and sets up input bitfields. This way the device driver tells the otherparts of the input systems what it is - what events can be generated oraccepted by this input device. Our example device can only generate EV_KEYtype events, and from those only BTN_0 event code. Thus we only set thesetwo bits. We could have used:
as well, but with more than single bits the first approach tends to beshorter.
Then the example driver registers the input device structure by calling:
This adds the button_dev structure to linked lists of the input driver andcalls device handler modules _connect functions to tell them a new inputdevice has appeared. input_register_device() may sleep and therefore mustnot be called from an interrupt or with a spinlock held.
While in use, the only used function of the driver is:
which upon every interrupt from the button checks its state and reports itvia the:
call to the input system. There is no need to check whether the interruptroutine isn’t reporting two same value events (press, press for example) tothe input system, because the input_report_* functions check thatthemselves.
Then there is the:
call to tell those who receive the events that we’ve sent a complete report.This doesn’t seem important in the one button case, but is quite importantfor for example mouse movement, where you don’t want the X and Y valuesto be interpreted separately, because that’d result in a different movement.
1.3. dev->open() and dev->close()¶
In case the driver has to repeatedly poll the device, because it doesn’thave an interrupt coming from it and the polling is too expensive to be doneall the time, or if the device uses a valuable resource (eg. interrupt), itcan use the open and close callback to know when it can stop polling orrelease the interrupt and when it must resume polling or grab the interruptagain. To do that, we would add this to our example driver:
Note that input core keeps track of number of users for the device andmakes sure that dev->open() is called only when the first user connectsto the device and that dev->close() is called when the very last userdisconnects. Calls to both callbacks are serialized.
The open() callback should return a 0 in case of success or any nonzero valuein case of failure. The close() callback (which is void) must always succeed.
1.4. Basic event types¶
The most simple event type is EV_KEY, which is used for keys and buttons.It’s reported to the input system via:
See uapi/linux/input-event-codes.h for the allowable values of code (from 0 toKEY_MAX). Value is interpreted as a truth value, ie any nonzero value means keypressed, zero value means key released. The input code generates events onlyin case the value is different from before.
In addition to EV_KEY, there are two more basic event types: EV_REL andEV_ABS. They are used for relative and absolute values supplied by thedevice. A relative value may be for example a mouse movement in the X axis.The mouse reports it as a relative difference from the last position,because it doesn’t have any absolute coordinate system to work in. Absoluteevents are namely for joysticks and digitizers - devices that do work in anabsolute coordinate systems.
Having the device report EV_REL buttons is as simple as with EV_KEY, simplyset the corresponding bits and call the:
function. Events are generated only for nonzero value.
However EV_ABS requires a little special care. Before callinginput_register_device, you have to fill additional fields in the input_devstruct for each absolute axis your device has. If our button device had alsothe ABS_X axis:
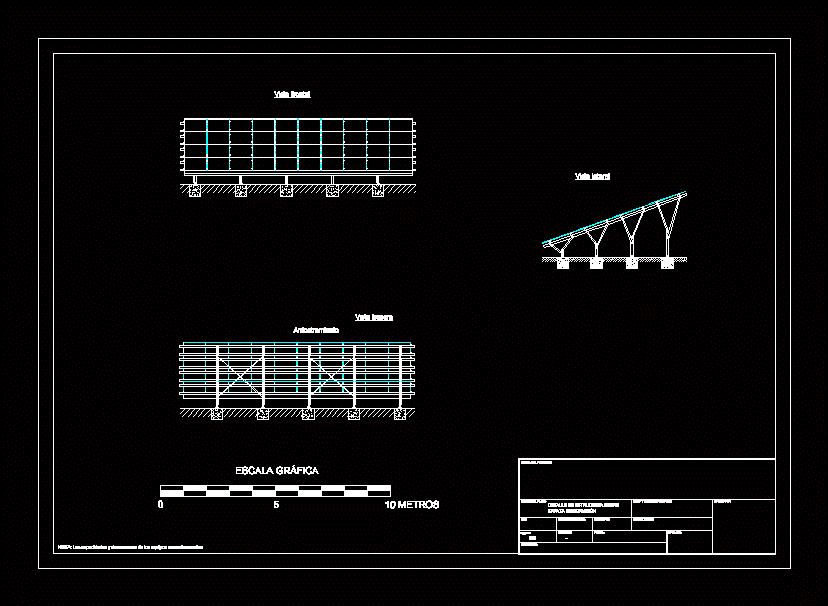
Or, you can just say:
This setting would be appropriate for a joystick X axis, with the minimum of0, maximum of 255 (which the joystick must be able to reach, no problem ifit sometimes reports more, but it must be able to always reach the min andmax values), with noise in the data up to +- 4, and with a center flatposition of size 8.
Drivers Ingenico Input Devices Software
If you don’t need absfuzz and absflat, you can set them to zero, which meanthat the thing is precise and always returns to exactly the center position(if it has any).
1.5. BITS_TO_LONGS(), BIT_WORD(), BIT_MASK()¶
These three macros from bitops.h help some bitfield computations:
1.6. The id* and name fields¶
The dev->name should be set before registering the input device by the inputdevice driver. It’s a string like ‘Generic button device’ containing auser friendly name of the device.
The id* fields contain the bus ID (PCI, USB, ...), vendor ID and device IDof the device. The bus IDs are defined in input.h. The vendor and device idsare defined in pci_ids.h, usb_ids.h and similar include files. These fieldsshould be set by the input device driver before registering it.
The idtype field can be used for specific information for the input devicedriver.
The id and name fields can be passed to userland via the evdev interface.
1.7. The keycode, keycodemax, keycodesize fields¶
These three fields should be used by input devices that have dense keymaps.The keycode is an array used to map from scancodes to input system keycodes.The keycode max should contain the size of the array and keycodesize thesize of each entry in it (in bytes).
Userspace can query and alter current scancode to keycode mappings usingEVIOCGKEYCODE and EVIOCSKEYCODE ioctls on corresponding evdev interface.When a device has all 3 aforementioned fields filled in, the driver mayrely on kernel’s default implementation of setting and querying keycodemappings.
1.8. dev->getkeycode() and dev->setkeycode()¶
getkeycode() and setkeycode() callbacks allow drivers to override defaultkeycode/keycodesize/keycodemax mapping mechanism provided by input coreand implement sparse keycode maps.
1.9. Key autorepeat¶
... is simple. It is handled by the input.c module. Hardware autorepeat isnot used, because it’s not present in many devices and even where it ispresent, it is broken sometimes (at keyboards: Toshiba notebooks). To enableautorepeat for your device, just set EV_REP in dev->evbit. All will behandled by the input system.
1.10. Other event types, handling output events¶
The other event types up to now are:
- EV_LED - used for the keyboard LEDs.
- EV_SND - used for keyboard beeps.
They are very similar to for example key events, but they go in the otherdirection - from the system to the input device driver. If your input devicedriver can handle these events, it has to set the respective bits in evbit,and also the callback routine:
This callback routine can be called from an interrupt or a BH (although thatisn’t a rule), and thus must not sleep, and must not take too long to finish.
The WinDriver™ 14.6.0 device driver development tool supports any device, regardless of its silicon vendor, and enables you to focus on your driver’s added-value functionality, instead of on the operating system internals. WinDriver’s driver development solution covers USB, PCI and PCI Express
Supported Operating Systems
Windows 10/8.1/Server 2016/Server 2012 R2/8/Server 2012/7/Server 2008 R2/Server 2008/Server 2003/XP, Embedded Windows 10/8.1/8/7, Windows CE/Mobile, and Linux- Easy user-mode driver development.
- Friendly DriverWizard allows hardware diagnostics without writing a single line of code.
- Automatically generates the driver code for the project in C, C#, Visual Basic .NET, Python, Java Delphi (Pascal), or Visual Basic 6.0.
- Supports any USB/PCI device, regardless of manufacturer.
- Enhanced support for specific chipsets frees the developer of the need to study the hardware’s specification.
- Applications are binary compatible across Windows 10/8.1/Server 2016/Server 2012 R2/8/Server 2012/7/Server 2008 R2/Server 2008/Server 2003/XP.
- Applications are source code compatible across all supported operating systems — Windows 10/8.1/Server 2016/Server 2012 R2/8/Server 2012/7/Server 2008 R2/Server 2008/Server 2003/XP, Embedded Windows 10/8.1/8/7, Windows CE (a.k.a. Windows Embedded Compact) 4.x–7.x (including Windows Mobile), and Linux.
- Can be used with common development environments, including MS Visual Studio, Borland C++ Builder, Borland Delphi, Visual Basic 6.0, MS eMbedded Visual C++, MS Platform Builder C++, GCC, Windows GCC, or any other appropriate compiler/environment.
- No WDK, ETK, DDI or any system-level programming knowledge required.
- Supports multiple CPUs.
- Includes dynamic driver loader.
- Comprehensive documentation and help files.
- Detailed examples in C, C#, Python, Java and VB.NET.
- HLK / HCK / WHQL certifiable driver (Windows).
- Two months of free technical support.
- No run-time fees or royalties.
WinDriver’s Top Features
- Fast: Shorten driver development cycle and time to market.
- Simple: No OS internals or kernel knowledge required.
- Stable: Field-tested on thousands of HW and OS configurations.
- Enhanced Chipset Support: Silicon partners libraries to jump-start your driver development. Special samples for various PCI/USB chip vendors, such as
Altera andXilinx . WinDriver generates a skeletal driver code, customized for the user’s hardware. - Multiple OS support and cross platform: WinDriver product line supports ?Windows 10/8.1/Server 2016/Server 2012 R2/8/Server 2012/7/Server 2008 R2/Server 2008/Server 2003/XP, Embedded Windows 10/8.1/8/7, Windows CE/Mobile, and Linux. The same driver will run under all supported operating systems without any code modifications. Just recompile!
- Immediate Hardware Access and Debugging: Test your hardware through a graphical user-mode application, without having to write a single line of code. Monitor kernel level activity throughout the driver development process.
- Performance Optimization: Use WinDriver’s Kernel PlugIn technology to develop your driver in the user-mode — then run performance critical sections of it in the Kernel Mode (Ring 0) to achieve optimal performance.
- HLK / HCK/ WHQL Certifiable Driver: WinDriver’s Windows drivers are WHQL ready — you will not need to invest time and efforts on making your driver WHQL compliant. Jungo also can prepare the WHQL submission package for you.
- Field-proven quality: WinDriver’s technology enables you to concentrate on your core business and successfully create first-rate drivers without having to invest redundant resources in driver development from scratch.
- User-mode programming: WinDriver’s architecture enables driver development in the user mode, while maintaining kernel-mode performance.
- 64-bit Support: Allow utilizing the additional bandwidth provided by 64-bit hardware and enable 64-bit data transfer on x86 platforms running 32-bit operating systems. Drivers developed with WinDriver will attain significantly better performance results than drivers written with the DDK or other driver development tools that do not support this feature.
- Complete .NET Framework Support: Easily incorporate WinDriver’s C# or VB.NET code into your existing .NET application using the powerful object oriented managed extensions for C++ library.
WinDriver products are accompanied with highly detailed technical references that are designed to assist you in various stages of the development process. If you have just started evaluating or using WinDriver, you may find our Quick Start Guideshelpful. Should you require more in-depth information, or would like to know more about the technical aspects of WinDriver, please refer to our Online Manual. For other technical resources, such as FAQs and technical documents — see WinDriver’s Support Page.
WinDriver for Servers/Data Centers
With Specific API for Server such as IPC, Buffer sharing, SRIOV and more. To enhance your WinDriver usage for you Server FPGA based devices.
WinDriver in your development product, API or SDK.
If you wish to use WinDriver within a development product, an API, or any part of a development product or environment you need to purchase the WinDriver SDK license.
Output Devices Of Computer
WinDriver support for Windows 10 IoT Core.
Starting WinDriver 12.5 WinDriver supports Windows 10 IoT Core x86, x64 and ARM.
Partners
WinDriver features a set of ready-made libraries and hardware access functions that provides enhanced support for our PCI/PCI Express and USB hardware Partners.
Read more about our Partners.
Drivers Ingenico Input Devices
Customers
WinDriver has thousands of customers worldwide that have used it to create numerous design wins. From scientific equipment to defense systems, from medical devices to consumer electronics, WinDriver assisted customers in focusing on their core expertise, by providing a straightforward yet reliable driver development solution.
See Also
WinDriver Latest Version:
14.6.0WinDriver Release notes
WinDriver Manuals:
USB
PCI/PCIe/ISA
Input Devices Of Computer
“When I found the Jungo Tools I initially looked at the online video showing how to drive the kit. When I tried out the real software I was amazed that within ten minutes I had my first driver working and could interact with my custom FPGA-based board.”Martin Kellermann | Staff Strategic Application Engineer| Xilinx
Drivers Ingenico Input Devices Device
Jungo’s professional services unit provides a complete Windows Hardware Certification (aka WHQL) submission service for
WinDriver customers.